Foreword
Sometimes you don’t want depend on some third party library, framework or component. Here’s step by step instruction how to embed Google Maps into Angular app using JavaScript API:
Setup
1. First of all you need to have your Google Maps API key.
2. In your project open up index.html
file and before
closing body tag insert Google Maps JavaScript API:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>My Awesome APP</title>
<base href="/">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="icon" type="image/x-icon" href="favicon.ico">
</head>
<body>
<ot-root></ot-root>
<!--Our JS API starts here-->
<script src="http://maps.googleapis.com/maps/api/js?key=YourGoogleMapsKey"></script>
<!--Our JS API ends here-->
</body>
</html>
3. On this step I would recommend to create a new component for the Google Maps to keep it’s logic in its own container. Here’s the exact logic of the GoogleMapComponent (Typescript file):
import { Component, OnInit } from '@angular/core';
declare const google: any;
@Component({
selector: 'app-google-map',
templateUrl: './google-map.component.html'
})
export class GoogleMapComponent implements OnInit {
constructor() { }
ngOnInit() {
let map;
let marker;
const DALLAS = {lat: 32.7767, lng: -96.7970};
map = new google.maps.Map(document.getElementById('map'), {
center: DALLAS,
zoom: 7
});
marker = new google.maps.Marker({
position: DALLAS,
map: map,
title: 'Hello World!'
});
}
}
Here’s a big guy: even though we’re in Typescript we can still write JavaScript logic because Typescript is superset of JavaScript!
4. In the template for this component (HTML file) lets add the following line:
<div id="map" class="google-map"></div>
Don’t forget to set explicit height to the google-map div! E.g. :
#map {
height: 500px;
}
5. The last step (the easiest one) you need to include your component into the parent template to show it. Here’s my result:
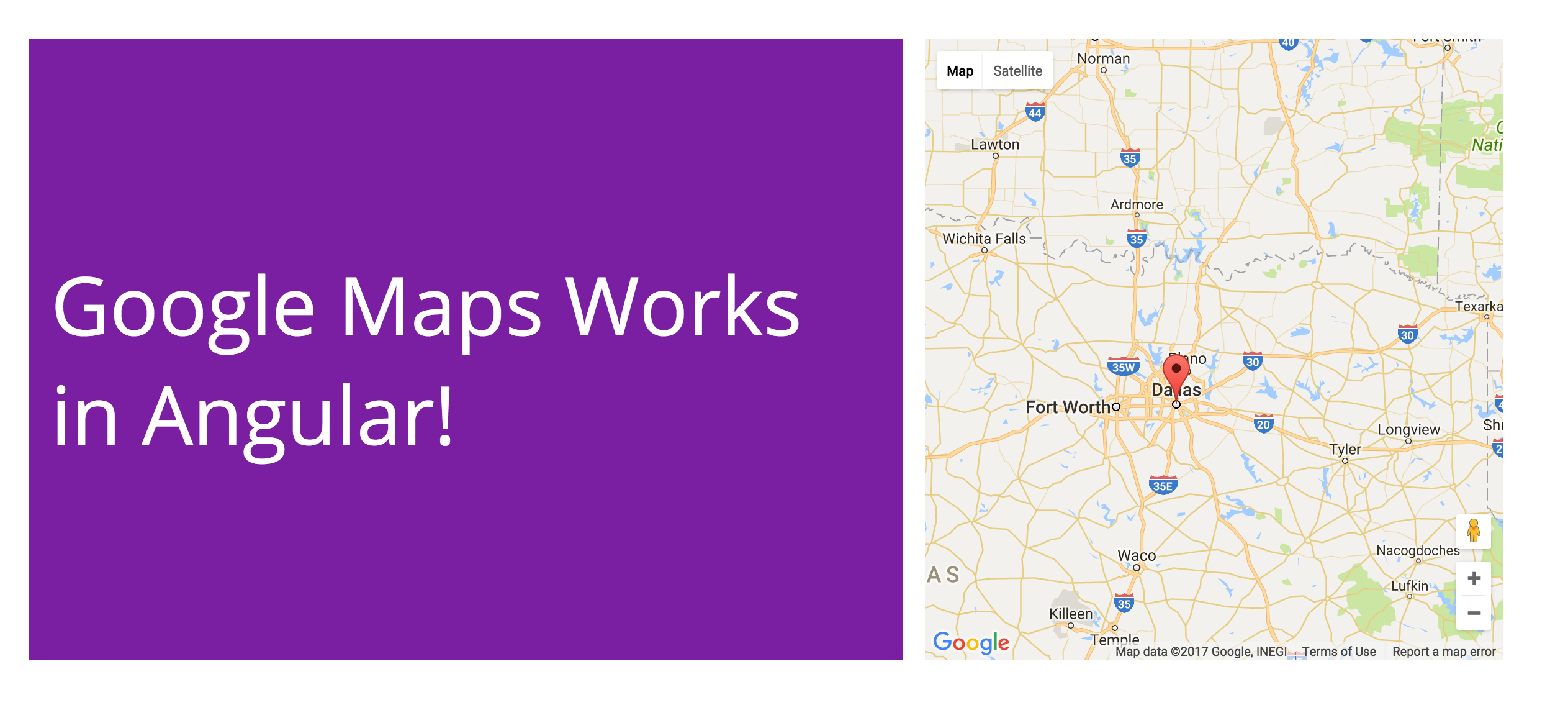
Conclusion
I described the basic idea how to integrate Angular with JavaScript API. For more details you can read Google’s official documentation regarding google maps.
In addition to that, you can apply this approach to any other JavaScript Libraries and API’s.